You typically create WCF proxy to run operations remotely. I came across a use case where I had to maximize the throughput to increase the number of transactions a single process can complete in a given time window. Yep, it was part of capacity planning to determine the number of hardware needed to complete business requirements (to be able to run N number of transactions in a given time window). So, one of the goals was to run everything in-proc (i.e. no remote calls) but use the same WCF proxy (which is used by others for out-of-proc calls). No change should be required for the client (minimum configuration change is okay).
Thanks to WCF because it forces the developers to use interfaces and you are step closer to SOA(knowingly or unknowingly). WCF is flexible (if you know what you are doing!) and it allows you to change the proxy behavior by simply overriding the Channel creation. I used CalculatorService wcf sample from Microsoft to proof the concept and it is here for you to utilize. You can find the complete solution here- SelfHost.zip.
private static readonly string assemblyFullName = "CalculatorService, Version=1.0.0.0, Culture=neutral, PublicKeyToken=214abdcc54e19955"; private static readonly string implementationClassName = "Microsoft.ServiceModel.Samples.Services.CalculatorService"; private static System.Reflection.Assembly assembly = null; protected override Microsoft.ServiceModel.Samples.Services.ICalculator CreateChannel() { Microsoft.ServiceModel.Samples.Services.ICalculator iCalculatorContract = null; bool executeLocally = false; bool.TryParse(System.Configuration.ConfigurationManager.AppSettings.Get("ExecuteLocally"), out executeLocally); if (executeLocally == false) { iCalculatorContract = base.CreateChannel(); } else { if (assembly == null) { assembly = System.Reflection.Assembly.Load(assemblyFullName); } System.Type type = assembly.GetType(implementationClassName); iCalculatorContract = System.Activator.CreateInstance(type) as Microsoft.ServiceModel.Samples.Services.ICalculator; } return iCalculatorContract; }
As you can see the changes are simple and you don’t touch any existing codes! Following images would provide you a quick view of the proxy and the client but you can download the complete source codes from this link SelfHost.zip. Just compile the solution, open the service EXE (to listen to port) and run the client. What to alter the proxy behavior? Just update the ExecuteLocally app setting to true.
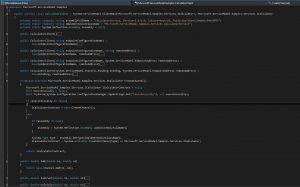
